📌 학습목표
- 서버에 대해 설명할 수 있다.
- Server와 Client의 구조를 설명할 수 있다.
- 서버와의 통신에 대해 설명할 수 있다.
- XML과 JSON에 대해 설명할 수 있다.
📝 Standard Mission
Retrofit2를 이용하여 앱에 OpenAPI 3개 이상 연동하기
- 사용 API는 자유, SDK 사용하지 않기
- JSON 형태의 OpenAPI 사용 권장
- 로그에서 받아온 값 출력하기
- 추천 사이트 : 공공데이터포털 (open.go.kr)
1️⃣ 영화진흥위원회 오픈 API
영화진흥위원회 오픈 API 중에 일일 박스오피스 API를 가져와볼 것이다.
영화진흥위원회 오픈API
제공서비스 영화관입장권통합전산망이 제공하는 오픈API서비스 모음입니다. 사용 가능한 서비스를 확인하고 서비스별 인터페이스 정보를 조회합니다.
www.kobis.or.kr
요청 인터페이스 필수는 key와 targetDt 두개이다.
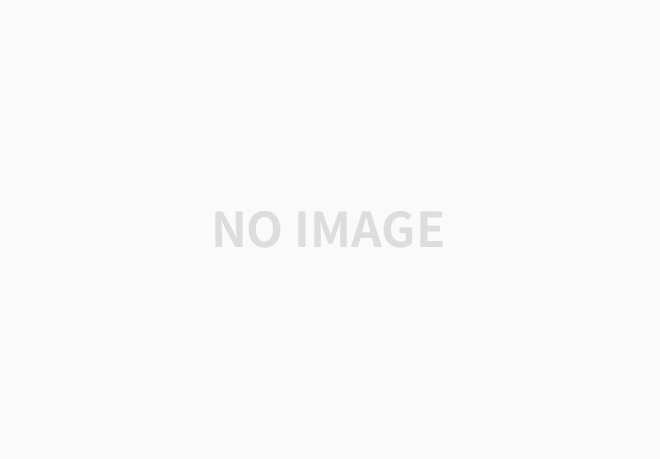
ex) json형태로 2012년 1월1일의 데이터를 받아오는 url
= http://kobis.or.kr/kobisopenapi/webservice/rest/boxoffice/searchDailyBoxOfficeList.json?key=f5eef3421c602c6cb7ea224104795888&targetDt=20120101
위 링크로 받아오는 응답 json을 살펴보면 다음과 같다. json에서 가져올 정보는
boxOfficeResult - dailyBoxOfficeList - {rank, movieNm}
으로 총 3개의 클래스를 만들어줘야한다.
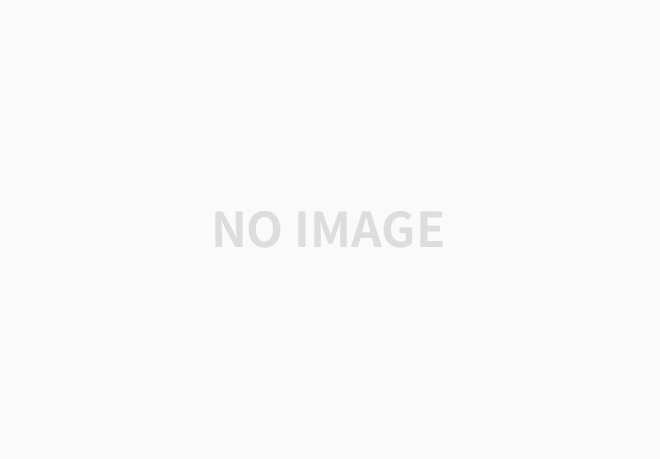
(1) boxOfficeResult 👉 MovieResponse.kt
@SerialzedName : JSON 에서 데이터에 매칭되는 이름 명시
package com.example.homework9
import com.google.gson.annotations.SerializedName
data class MovieResponse(
@SerializedName("boxOfficeResult")
var boxofficeResult: BoxOfficeResult?
)
(2) dailyBoxOfficeList 👉 BoxOfficeResult.kt
package com.example.homework9
import com.google.gson.annotations.SerializedName
data class BoxOfficeResult(
@SerializedName("dailyBoxOfficeList")
var dailyBoxOfficeList: List<MovieDto> = arrayListOf()
//받아온 결과를 MovieDto list 형태로 만든다.
)
(3) rank & movieNm 👉 MovieDto.kt
package com.example.homework9
import com.google.gson.annotations.SerializedName
import java.io.Serializable
data class MovieDto(
@SerializedName("rank")
var rank: String?
@SerializedName("movieNm")
var movieNm: String?,
) : Serializable {
}
MovieAPI.kt
마지막으로, json 결과를 받아올 retrofit 통신 인터페이스를 생성한다.
package com.example.homework9
import retrofit2.Call
import retrofit2.http.GET
import retrofit2.http.Query
interface MovieAPI {
@GET("kobisopenapi/webservice/rest/boxoffice/searchDailyBoxOfficeList.json")
fun getBoxOffice(
@Query("key") key: String,
@Query("targetDt") targetDt: String,
): Call<MovieResponse> // api 호출 MovieResponse 형태로 받을 예정
}
MainActivity.kt
retorofit client 파일을 만들어주고 MainActivity에서 정보가 제대로 넘어오는지 Log를 찍어봤다.
(아래 코드에서 API Key는 가렸다)
package com.example.homework9
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.util.Log
import com.example.homework9.databinding.ActivityMainBinding
import retrofit2.Call
import retrofit2.Callback
import retrofit2.Retrofit
import retrofit2.converter.gson.GsonConverterFactory
class MainActivity : AppCompatActivity() {
private lateinit var binding: ActivityMainBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityMainBinding.inflate(layoutInflater)
setContentView(binding.root)
// 웹 브라우저 창 열기
val retrofit = Retrofit.Builder()
.baseUrl("https://www.kobis.or.kr/")
// json 형태로 들어오면 Gson 라이브러리에서 우리가 사용할 수 있는 객체 형태로 바꿔준다.
.addConverterFactory(GsonConverterFactory.create())
.build() // Retrofit 객체 생성
// 어떤 주소를 입력
val MovieAPI = retrofit.create(MovieAPI::class.java)
// 입력한 주소 중에 하나로 연결 시도
// 네트워크 통신이다 보니 비동기적으로 작동해야 돼서 queue 에 넣는 것. 백그라운드에서 작동
MovieAPI.getBoxOffice("API KEY", "20221126").enqueue(object: Callback<MovieResponse> {
// 응답 받았을 때
override fun onResponse(call: Call<MovieResponse>, response: retrofit2.Response<MovieResponse>) {
val responseData = response.body()
// 데이터가 안 넘어올 수 있어서 체크
if(responseData != null) {
val list : List<MovieDto> = responseData!!.boxofficeResult!!.dailyBoxOfficeList
Log.d("Retrofit", "Response\nMovie: ${list}")
}
// 응답이 왔지만 실패인 경우
else {
// http 프로토콜 오류 code (전세계 규격 코드)
Log.w("Retrofit", "Response Not Successful ${response.code()}")
}
}
// 서버와 연결을 실패했을 때
override fun onFailure(call: Call<MovieResponse>, t: Throwable) {
Log.e("Retrofit", "Error!", t)
}
})
}
}
MovieDto에 List 형태로 담겨서 잘 출력되는 것을 확인할 수 있다.
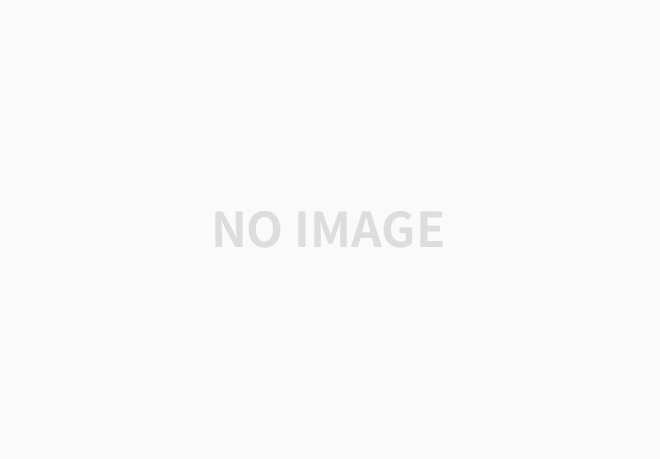

2️⃣ 날씨 오픈 API
Current weather data - OpenWeatherMap
Access current weather data for any location on Earth including over 200,000 cities! We collect and process weather data from different sources such as global and local weather models, satellites, radars and a vast network of weather stations. Data is avai
openweathermap.org
요청 인터페이스 필수는 lat, lon와 appid다.
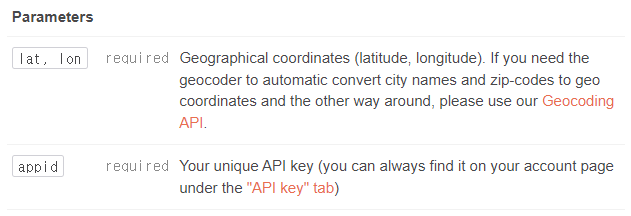
ex) json형태로 위도44.34 경도 10.99인 위치의 날씨
= https://api.openweathermap.org/data/2.5/weather?lat=44.34&lon=10.99&appid={api_key}
위 링크로 받아오는 응답 json을 살펴보면 다음과 같다. json에서 가져올 정보는
weather - {main, icon}
으로 총 2개의 클래스를 만들어줘야한다.
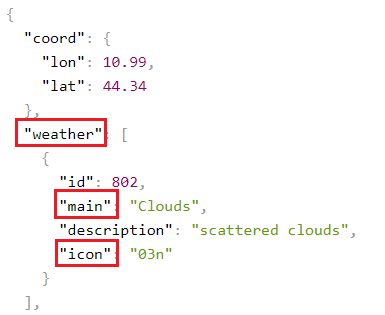
(1) weather 👉 WeatherResponse.kt
package com.example.homework9
import com.google.gson.annotations.SerializedName
data class WeatherResponse(
@SerializedName("weather")
var weather: List<WeatherDto> = arrayListOf()
)
(2) {main, icon} 👉 WeatherDto.kt
package com.example.homework9
import com.google.gson.annotations.SerializedName
import java.io.Serializable
data class WeatherDto(
@SerializedName("main")
var main: String?,
@SerializedName("icon")
var icon: String?,
) : Serializable {
}
WeatherAPI.kt
마지막으로, json 결과를 받아올 retrofit 통신 인터페이스를 생성한다.
package com.example.homework9
import retrofit2.Call
import retrofit2.http.GET
import retrofit2.http.Query
interface WeatherAPI {
@GET("weather?")
fun getWeather(
@Query("lat") lat: Int,
@Query("lon") lon: Int,
@Query("appid") appid: String,
): Call<WeatherResponse>
}
MainActivity.kt
retorofit client 파일을 만들어주고 MainActivity에서 정보가 제대로 넘어오는지 Log를 찍어봤다.
(아래 코드에서 API Key는 가렸다)
package com.example.homework9
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.util.Log
import com.example.homework9.databinding.ActivityMainBinding
import retrofit2.Call
import retrofit2.Callback
import retrofit2.Retrofit
import retrofit2.converter.gson.GsonConverterFactory
class MainActivity : AppCompatActivity() {
private lateinit var binding: ActivityMainBinding
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
binding = ActivityMainBinding.inflate(layoutInflater)
setContentView(binding.root)
val retrofit = Retrofit.Builder()
.baseUrl("https://api.openweathermap.org/data/2.5/")
.addConverterFactory(GsonConverterFactory.create())
.build()
val WeatherAPI = retrofit.create(WeatherAPI::class.java)
WeatherAPI.getWeather(37, 126, "API KEY").enqueue(object: Callback<WeatherResponse> {
override fun onResponse(call: Call<WeatherResponse>, response: retrofit2.Response<WeatherResponse>) {
val responseData = response.body()
if(responseData != null) {
val list : List<WeatherDto> = responseData!!.weather!!
Log.d("Retrofit", "Response\nWeather: ${list}")
}
else {
Log.w("Retrofit", "Response Not Successful ${response.code()}")
}
}
override fun onFailure(call: Call<WeatherResponse>, t: Throwable) {
Log.e("Retrofit", "Error!", t)
}
})
}
}
서울의 위도와 경도를 통해 날씨와 온도를 불러왔다!

'🍞 대외활동 > Univ Makeus Challenge' 카테고리의 다른 글
[데모데이] 3rd UMC DemoDay 회고 (0) | 2023.02.17 |
---|---|
[UMC] Android 9주차 워크북 (Network) - 소셜 로그인 (0) | 2022.11.28 |
[UMC] 3rd UMC 해커톤 회고 (0) | 2022.11.22 |
[UMC] Android 8주차 워크북 (Database) (0) | 2022.11.21 |
[UMC] Android 7주차 워크북 (Thread) (0) | 2022.11.17 |