BMI을 계산하기 위해서 Kotlin 문법을 사용해 BMI 계산기를 만들어볼 것이다.
Layout을 그리는 법
- LinearLayout 사용하기
- TextView의 속성들과 사용하는 법
- EditText의 속성들과 사용하는 법
- Button 사용하는 법
📌 알게 된 점
1. EditText에 inputType을 number로 주면 숫자 키패드 자판이 나온다.
2. 안드로이드 핸드폰에 따라 해상도와 화면 크기가 다르기 때문에 일정하게 하기 위해 dp를 사용한다.
3. 사용자에 따라 글씨를 크게 키우고 싶을 수도 있으므로 글씨 크기는 sp로 설정한다.
4. 코드 정렬을 하기 위해서 Reformat code(Ctrl+Alt+L)라는 단축키를 사용한다.
5. MainActivity에서 ResultActivity로 데이터를 넘겨주기 위해선 intent를 사용한다.
데이터를 putExtra로 보내주고 getExtra로 받는다.
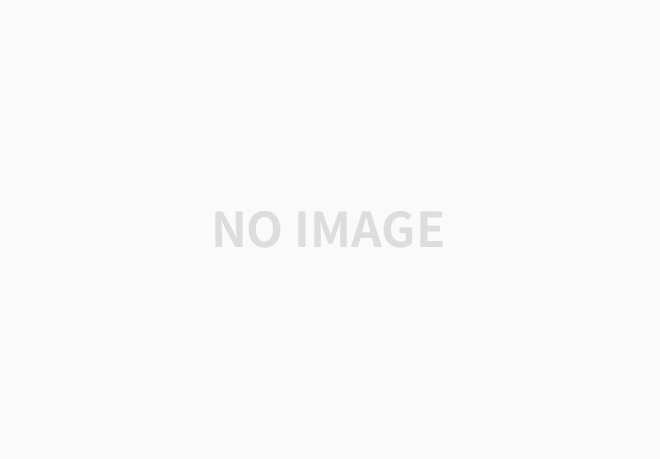
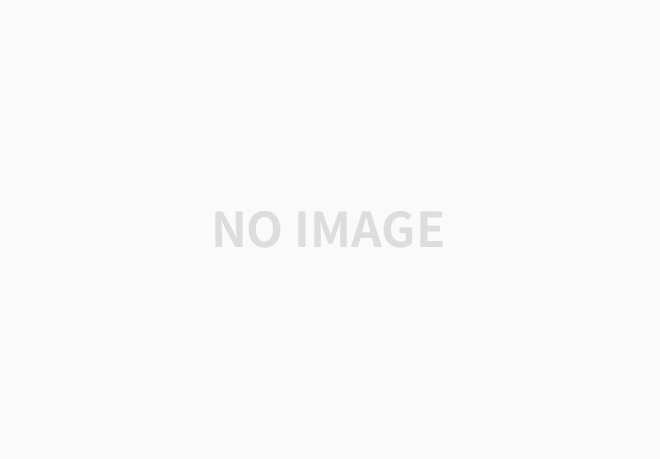
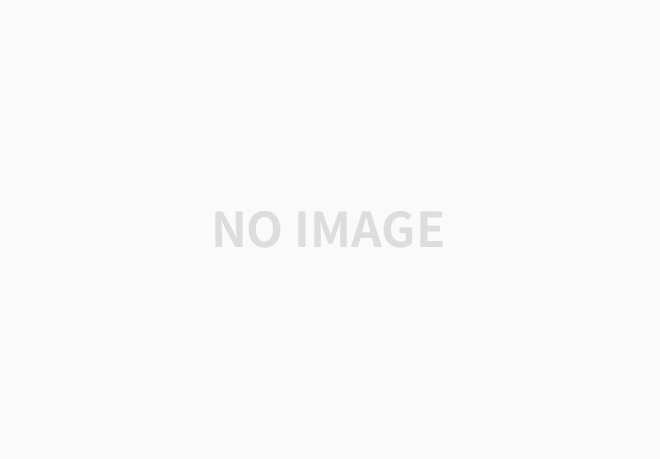
인텐트 및 인텐트 필터 | Android 개발자 | Android Developers
An Intent is a messaging object you can use to request an action from another app component . Although intents facilitate communication between components in several ways, there are three fundamental use cases: An Activity represents a single screen in…
developer.android.com
📌 최종 코드
- Activity
MainActivity.kt
package com.example.bmi_calculator
import android.content.Intent
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.util.Log
import android.widget.Button
import android.widget.EditText
import android.widget.Toast
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// R이 주소값
setContentView(R.layout.activity_main)
val heightEditText: EditText = findViewById(R.id.heightEditText) // type 명시
val weightEditText = findViewById<EditText>(R.id.weightEditText) // findViewById 반환하면서 type 추론
val resultButton = findViewById<Button>(R.id.resultButton)
resultButton.setOnClickListener {
// 비어있으면 앱이 종료되기 때문에 예외처리
if(heightEditText.text.isEmpty() || weightEditText.text.isEmpty()) {
Toast.makeText(this, "빈 값이 있습니다", Toast.LENGTH_SHORT)
// 함수가 중첩되어있기 때문에 어느 함수에서 return 할 것인지 명시
return@setOnClickListener
}
// String 에서 Int 로 바꿔줘야함
val height: Int = heightEditText.text.toString().toInt()
val weight: Int = weightEditText.text.toString().toInt()
val intent = Intent(this, ResultActivity::class.java)
intent.putExtra("height", height)
intent.putExtra("weight", weight)
startActivity(intent)
}
}
}
ResultActivity.kt
package com.example.bmi_calculator
import android.os.Bundle
import android.util.Log
import android.widget.TextView
import androidx.appcompat.app.AppCompatActivity
import kotlin.math.pow
class ResultActivity: AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_result)
val height = intent.getIntExtra("height", 0)
val weight = intent.getIntExtra("weight", 0)
val bmi = weight / (height / 100.0).pow(2.0)
val resultText = when {
bmi >= 35.0 -> "고도 비만"
bmi >= 30.0 -> "중정도 비만"
bmi >= 25.0 -> "경도 비만"
bmi >= 23.0 -> "과제충"
bmi >= 18.5 -> "정상체중"
else -> "저체중"
}
val resultValueTextView = findViewById<TextView>(R.id.bmiResultTextView)
val resultStringTextView = findViewById<TextView>(R.id.resultTextView)
resultValueTextView.text = bmi.toString()
resultStringTextView.text = resultText
}
}
- Layout
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:padding="16dp"
tools:context=".MainActivity">
<TextView
android:id="@+id/textView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@string/height"
android:textColor="@color/custom_black"
android:textSize="20sp"
android:textStyle="bold" />
<EditText
android:id="@+id/heightEditText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:inputType="number" />
<TextView
android:id="@+id/textView2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="30dp"
android:text="@string/weight"
android:textColor="@color/custom_black"
android:textSize="20sp"
android:textStyle="bold" />
<EditText
android:id="@+id/weightEditText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:inputType="number" />
<Button
android:id="@+id/resultButton"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="30dp"
android:text="확인하기" />
</LinearLayout>
activity_result.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="10dp"
android:gravity="center"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="20dp"
tools:text="BMI: " />
<TextView
android:id="@+id/bmiResultTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="20dp"
tools:text="23.11111111" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="20dp"
tools:text="결과는 " />
<TextView
android:id="@+id/resultTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="20dp"
tools:text="과제중입니다." />
</LinearLayout>
</LinearLayout>
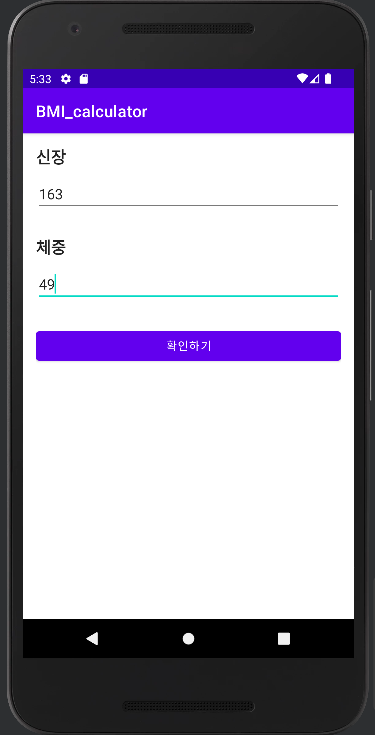
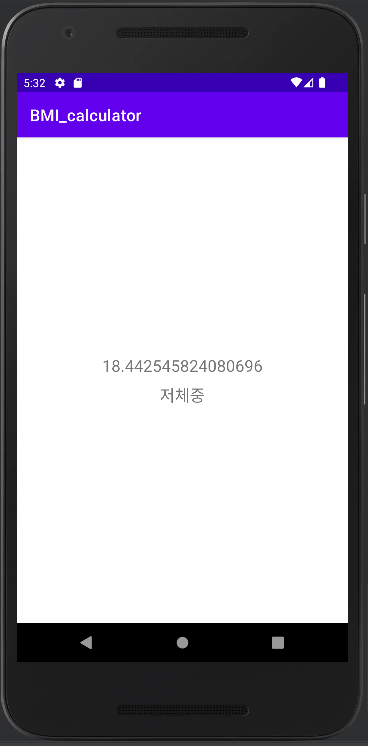
'🍞 Front-End > Android' 카테고리의 다른 글
[Android] 비밀 다이어리 (0) | 2022.10.28 |
---|---|
[Android] 로또 번호 추첨기 (2) | 2022.10.27 |
[Android] 안드로이드 리사이클러뷰(RecyclerView) (0) | 2022.10.23 |
[Android] No speakable text present 경고 없애기 (0) | 2022.10.18 |
[Kotlin] 안드로이드 스튜디오 AVD(Android Virtual Device) 설정 (0) | 2022.09.22 |